Identifying mobile devices has always been a critical element in the development of applications, as well as for software and websites. This is crucial for various reasons, especially to tailor a distinct user experience for mobile users.
The UAParser.js library, with millions of weekly package downloads, is often sufficient when building something from the ground up. However, exploring alternative methods for detecting mobile browsers using JavaScript can offer simplicity without relying on external libraries.
In this article, I will present several JavaScript techniques for detecting mobile users, providing straightforward solutions for the task. Feel free to let me know if I've overlooked any methods!
navigator.userAgent
The holy grail of browser detection is the navigator.UserAgent property.
if (/Android|iPhone/i.test(navigator.userAgent)) { // This checks if the current device is in fact mobile } // an alternative structure to check individual matches if ( navigator.userAgent.match(/Android/i) || navigator.userAgent.match(/iPhone/i) ) { // making individual checks }
This is, of course, a very primitive way of doing it. It can easily be manipulated as the User-Agent property can be spoofed. But, you can still use it in various projects because it does the job. E.g. Landing pages or making a custom redirect to a mobile version.
How to display a warning message if the user uses an unsupported or outdated browser?
One way this property can be used is to check whether or not the user is using a specific version of a browser, and, if not - perform an action.
In this example, it's a simple alert message:
// get the user agent string and extract the browser version var userAgent = navigator.userAgent; var browserVersion = userAgent.substring(userAgent.indexOf("Chrome") + 7); // check if the browser version is less than the minimum supported version if (parseFloat(browserVersion) < 107) { // show a warning message alert("Your browser is outdated and not supported. Please upgrade to the latest version."); }
☰ What does the "+ 7" do?
How to redirect users to a mobile version of the site if they're browsing from mobile?
To detect mobile devices and serve a mobile-optimized version of the website, you can use the navigator.userAgent
property to check for common mobile user agents. For example:
// check for common mobile user agents if (navigator.userAgent.match(/Android/i) || navigator.userAgent.match(/webOS/i) || navigator.userAgent.match(/iPhone/i) || navigator.userAgent.match(/iPad/i) || navigator.userAgent.match(/iPod/i) || navigator.userAgent.match(/BlackBerry/i) || navigator.userAgent.match(/Windows Phone/i)) { // the user is using a mobile device, so redirect to the mobile version of the website window.location = "https://www.yoursite.com/mobile"; }
How to use navigator.userAgent
to detect browser type and display content based on the specific browser?
Sure, in a particular scenario, you might have a need to identify a particular web browser, like distinguishing between Chrome and Firefox (or in this instance, between Android and iPhone browsers). This information can then be utilized to tailor the displayed content accordingly. This technique is commonly employed to offer or alter download links based on the user's browser type.
In such a case, you can use the following function.
function detectBrowser() { let userAgent = navigator.userAgent; let browserName; if (userAgent.match(/chrome|chromium|crios/i)) { browserName = "Chrome"; } else if (userAgent.match(/firefox|fxios/i)) { browserName = "Firefox"; } else if (userAgent.match(/safari/i)) { browserName = "Safari"; } else if (userAgent.match(/opr\//i)) { browserName = "Opera"; } else if (userAgent.match(/edg/i)) { browserName = "Edge"; } else if (userAgent.match(/android/i)) { browserName = "Android"; } else if (userAgent.match(/iphone/i)) { browserName = "iPhone"; } else { browserName = "Unknown"; } document.querySelector("div.form-style h3").innerText = "You are browsing with: " + browserName + ""; }
You are browsing with: Firefox
You can comment out the various else if
statements for the browsers you don't want to check, and likewise - you can add additional browsers you do want to check.
TouchEvent
One method to detect mobile users is to check if the device has a touch screen.
Using the GlobalEventHandlers.ontouchstart property you can make a simple check to see how the user interacted with your app. If the interaction came from a touch screen, you can then return a mobile version of the app or page.
if ("ontouchstart" in document.documentElement) { // content for touch-screen (mobile) devices } else { // everything else (desktop) }
Touch-screen devices like Surface do not have this property. So, users coming from desktop-based touch devices will still see the desktop version of your pages.
Window.matchMedia()
The Window.matchMedia() is one of the best properties for detecting mobile users with JavaScript. And it is so because it lets you interact with CSS directly.
In a lot of cases, media queries are superior because they have built-in mobile detection tools. For example, you can make a call to check if “pointer:coarse” is true.
This specific statement validates whether the device’s pointer is fine or coarse.
let isMobile = window.matchMedia("(pointer:coarse)").matches;
Alternatively, the device might have both a fine and coarse pointer. For this use case, we can check if any pointers are coarse.
let isMobile = window.matchMedia("(any-pointer:coarse)").matches;
Keep in mind that this only validates the query as true or false. A more refined way to check for mobile devices is to use media queries directly.
let isMobile = window.matchMedia("only screen and (max-width: 480px)").matches;
This query will directly check the max-width of the device and assert whether it matches the criteria. Again, this is quite a lot of work for getting all devices correctly. As such, it’s easier to use a pre-built library with all the device types already defined.
Useful in certain contexts
Over time, numerous JavaScript properties designed for detecting device types have emerged. While some have become deprecated, many others have been incorporated into libraries. Interestingly, leveraging libraries has proven to be the most effective approach for accurate mobile detection.
Additionally, contemporary frameworks often come equipped with built-in mobile detection features. Exploring these capabilities can be advantageous, especially if you prefer not to undertake the manual effort of implementing device detection mechanisms.
Libraries for Detecting Mobile Devices
This section provides an overview of widely used JavaScript libraries designed for identifying mobile devices. It's crucial to note that these libraries are specifically tailored for JavaScript. For accurate integration into your application, it is recommended to consult the documentation associated with each library.
UAParser.js
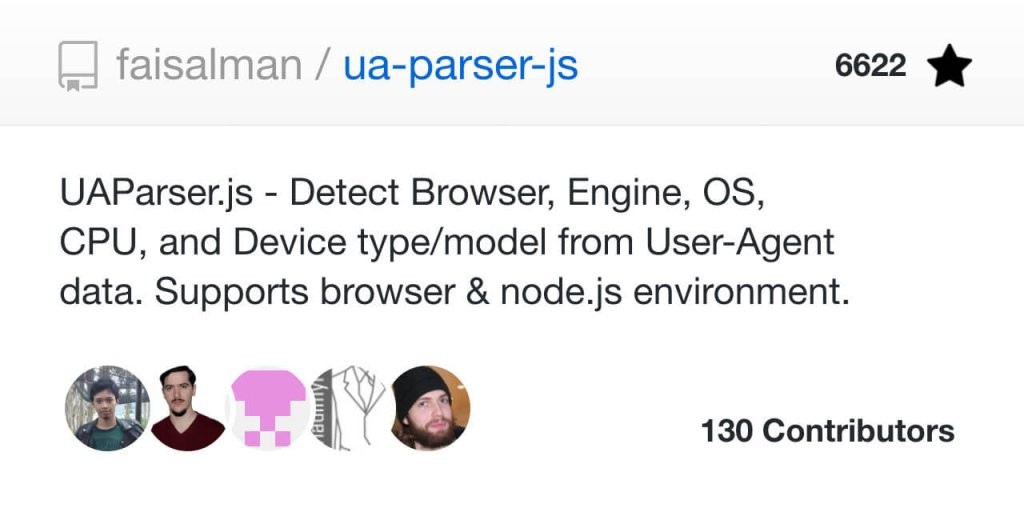
As far as complete libraries go, UAParser is the best there is. With more than 10 million weekly downloads on npm alone - UAParser is the defacto solution for detecting mobile devices. As the name gives it away - the library works by parsing User-Agent strings.
However, what makes it so popular is the fact that you can parse hundreds of device variations. And, all of it is very well documented. You can go from practical device vendors to more intricate detection patterns like CPU architecture.
mobile-detect.js
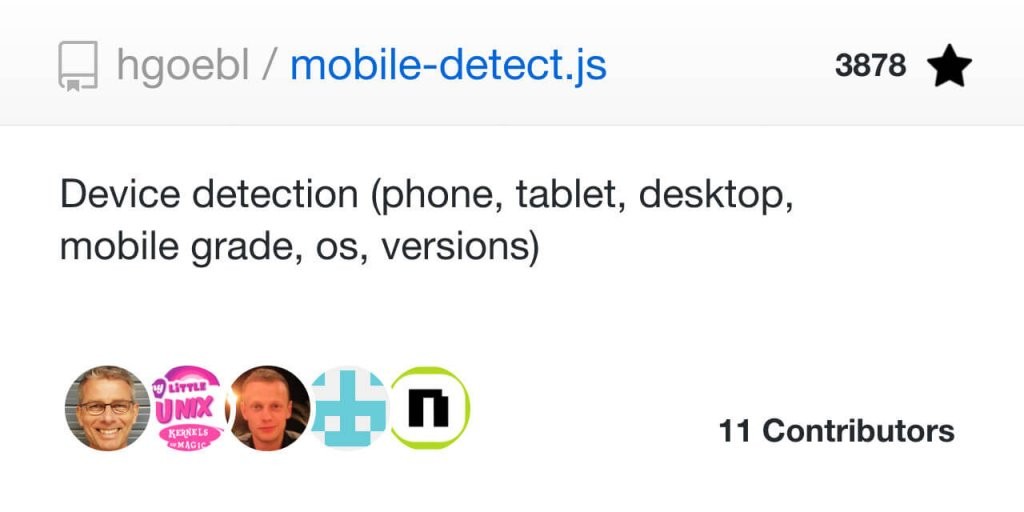
This is a fairly straightforward port of the Mobile Detect library for PHP, provided to the community by Heinrich Goebl. The library itself uses User-Agent for detection, so as we discussed earlier - not the best option.
Still, it should do the job when it comes to practical HTML templates or portfolio projects.
isMobile
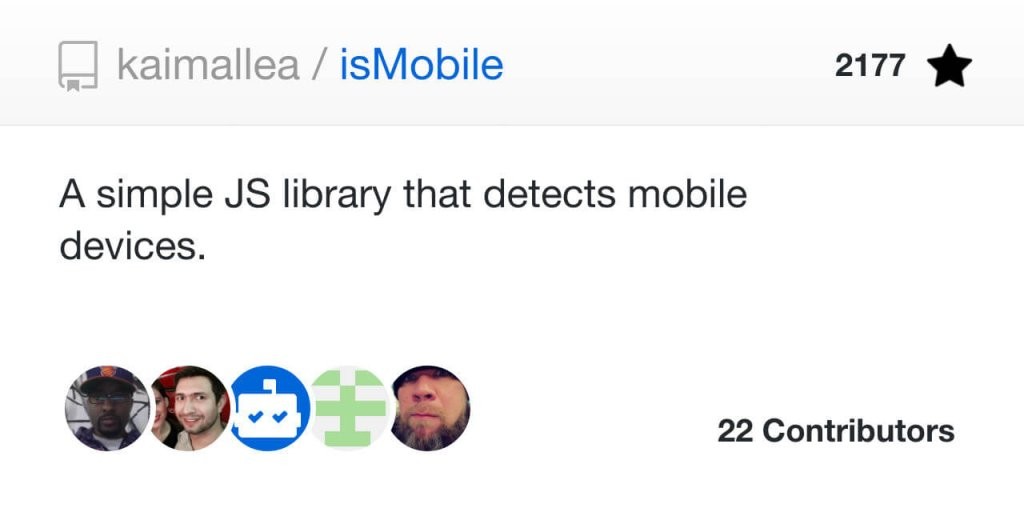
Here we have another take on the User-Agent Navigator property from Kai Mallea. While still a simplistic solution, I like that isMobile provides a variety of specifications. For example, you can test for any mobile devices or specific ones like phone or tablet.
react-device-detect
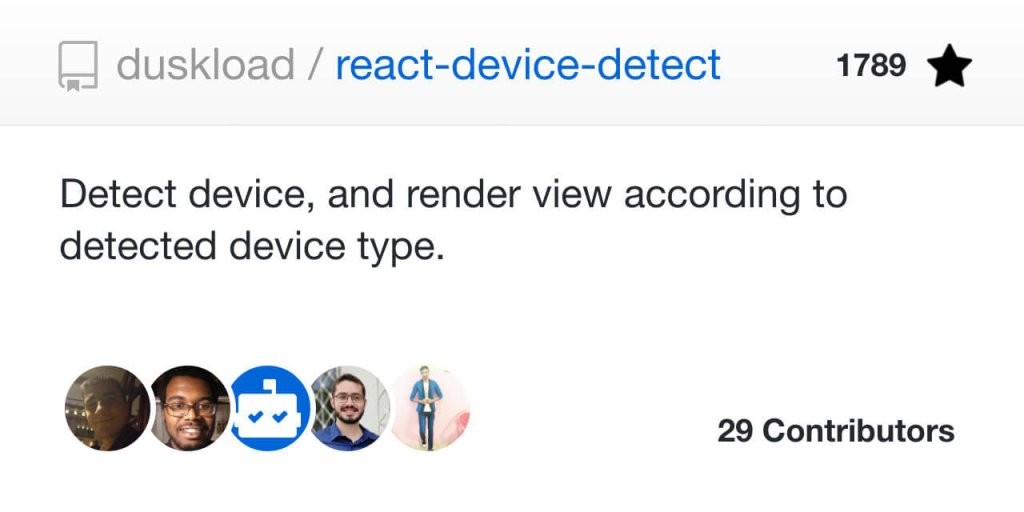
Are you a React.js developer?
If you're looking for a solution, Michael Laktionov's library might be just what you need. The library operates intuitively: it identifies the device type and then generates the view accordingly. Seamless integration with components is ensured, and the library offers additional customization options through API calls.
A notable feature is the extensive range of selectors incorporated into this library. It encompasses devices ranging from smart TVs and wearables to various iPhone models, providing a wide array of design options for developing applications tailored to specific devices.
No comments:
Post a Comment